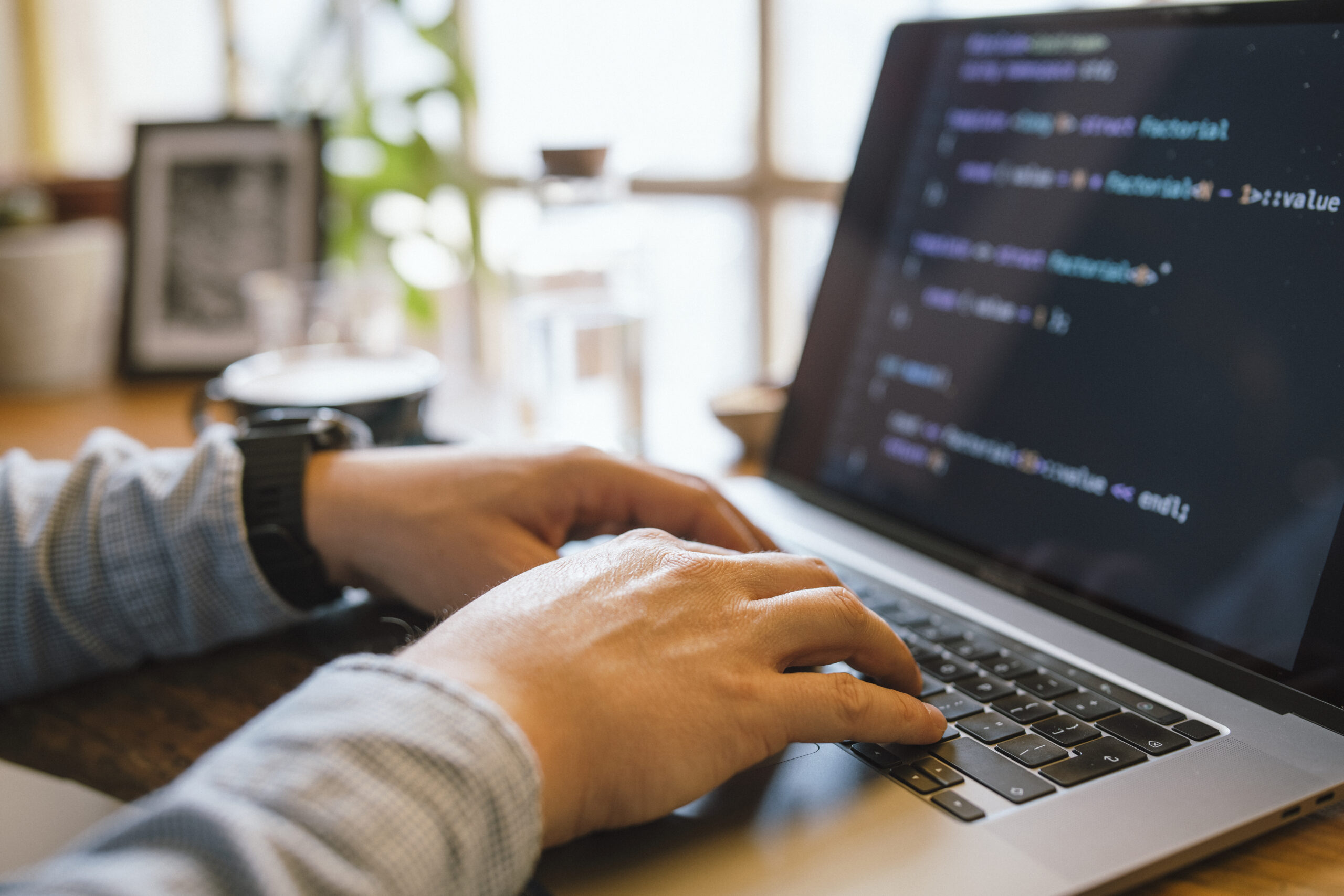
Debugging is Probably the most necessary — however usually neglected — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productivity. Here are several procedures that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest means builders can elevate their debugging expertise is by mastering the resources they use every day. Though producing code is one particular Portion of improvement, knowing tips on how to communicate with it successfully during execution is Similarly crucial. Modern progress environments arrive equipped with highly effective debugging capabilities — but lots of builders only scratch the surface of what these instruments can do.
Consider, such as, an Integrated Advancement Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code about the fly. When used effectively, they let you notice exactly how your code behaves through execution, that is priceless for tracking down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor network requests, perspective actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can flip annoying UI issues into manageable jobs.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about running processes and memory management. Mastering these applications might have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into comfortable with version Handle programs like Git to be familiar with code history, discover the exact second bugs have been launched, and isolate problematic alterations.
In the long run, mastering your applications means going beyond default settings and shortcuts — it’s about creating an personal familiarity with your progress ecosystem so that when issues crop up, you’re not lost in the dark. The better you know your tools, the more time you can spend resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
The most vital — and often ignored — steps in helpful debugging is reproducing the condition. In advance of jumping in to the code or making guesses, builders need to have to make a constant environment or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug turns into a recreation of opportunity, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Check with queries like: What steps resulted in the issue? Which natural environment was it in — advancement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it gets to be to isolate the precise circumstances less than which the bug occurs.
As you’ve collected more than enough data, attempt to recreate the situation in your local setting. This might necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The problem seems intermittently, think about producing automated exams that replicate the sting cases or state transitions included. These checks not just support expose the problem but in addition protect against regressions in the future.
At times, The difficulty may be surroundings-precise — it'd occur only on specified functioning systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It requires patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. Which has a reproducible scenario, you can use your debugging resources a lot more efficiently, take a look at probable fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders thrive.
Go through and Comprehend the Error Messages
Mistake messages will often be the most respected clues a developer has when some thing goes wrong. Rather then looking at them as irritating interruptions, developers should really study to deal with error messages as immediate communications with the process. They usually tell you exactly what transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by examining the concept cautiously As well as in entire. Numerous builders, particularly when under time force, glance at the main line and quickly begin earning assumptions. But deeper in the mistake stack or logs might lie the genuine root result in. Don’t just duplicate and paste error messages into search engines — examine and realize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line selection? What module or operate brought on it? These concerns can tutorial your investigation and stage you towards the liable code.
It’s also beneficial to be aware of the terminology from the programming language or framework you’re working with. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable styles, and Understanding to acknowledge these can drastically quicken your debugging course of action.
Some errors are vague or generic, and in Those people circumstances, it’s important to look at the context by which the error transpired. Verify relevant log entries, enter values, and up to date changes inside the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger concerns and supply hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective instruments inside of a developer’s debugging toolkit. When made use of effectively, it provides real-time insights into how an application behaves, helping you understand what’s happening underneath the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Widespread logging amounts contain DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic details throughout improvement, INFO for typical gatherings (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for true issues, and FATAL in the event the technique can’t proceed.
Steer clear of flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Focus on vital functions, state improvements, input/output values, and important determination points as part of your code.
Format your log messages Evidently and constantly. Include context, for example timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging tactic, you are able to decrease the time it's going to take to spot concerns, get further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To proficiently identify and repair bugs, developers have to tactic the procedure like a detective solving a mystery. This attitude will help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or general performance challenges. Just like a detective surveys against the law scene, obtain just as much applicable information and facts as you can without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a clear image of what’s happening.
Next, form hypotheses. Talk to you: What may very well be resulting in this habits? Have any changes lately been made to the codebase? Has this issue happened prior to under identical situation? The purpose is usually to narrow down possibilities and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble inside a managed natural environment. In case you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code concerns and Enable the final results lead you nearer to the truth.
Pay back near attention to smaller particulars. Bugs normally conceal in the minimum anticipated sites—just like a lacking semicolon, an off-by-a single mistake, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly comprehending it. Non permanent fixes might cover the real dilemma, just for it to resurface later.
And lastly, keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming problems and enable others realize your reasoning.
By wondering like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed problems in sophisticated units.
Create Exams
Producing checks is one of the most effective approaches to transform your debugging competencies and overall improvement effectiveness. Exams not merely enable capture bugs early but will also function a security Web that offers you self-confidence when creating adjustments to the codebase. A properly-examined software is simpler to debug since it lets you pinpoint particularly wherever and when a challenge takes place.
Begin with device checks, which deal with unique capabilities or modules. These smaller, isolated checks can promptly expose no matter if a certain piece of logic is Functioning as anticipated. When a test fails, you straight away know where by to glimpse, noticeably cutting down enough time put in debugging. Unit checks are In particular handy for catching regression bugs—troubles that reappear right after previously remaining fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These assistance be certain that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If a little something breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Producing exams also forces you to definitely Feel critically regarding your code. To test a aspect effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging an issue, producing a failing test that reproduces the bug could be a robust first step. After the take a look at fails get more info regularly, it is possible to focus on fixing the bug and look at your exam pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing recreation into a structured and predictable course of action—helping you catch a lot more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging a tricky problem, it’s effortless to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your thoughts, decrease disappointment, and sometimes see The problem from a new perspective.
When you're as well close to the code for too long, cognitive tiredness sets in. You could commence overlooking clear mistakes or misreading code which you wrote just hours earlier. In this point out, your Mind will become considerably less productive at difficulty-solving. A brief wander, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid prevent burnout, Primarily through for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping away enables you to return with renewed Electrical power and also a clearer attitude. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–60 minutes, then have a 5–ten minute split. Use that point to move all over, stretch, or do a thing unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to a lot quicker and more effective debugging In the long term.
In brief, getting breaks is not a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your standpoint, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each one can teach you some thing worthwhile when you go to the trouble to reflect and analyze what went Improper.
Commence by inquiring by yourself some vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it happen to be caught earlier with far better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or understanding and help you build stronger coding habits going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you note down bugs you’ve encountered, the way you solved them, and Anything you figured out. After a while, you’ll start to see patterns—recurring challenges or popular mistakes—you could proactively prevent.
In staff environments, sharing Whatever you've discovered from the bug with the peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short write-up, or A fast information-sharing session, assisting others stay away from the identical issue boosts staff effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as vital parts of your growth journey. In the end, many of the greatest builders aren't those who create best code, but those that repeatedly learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and able developer. The next time you are knee-deep in a very mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.